MyBatis 3.3.0 Interview Questions and Answers: Prepare for Success
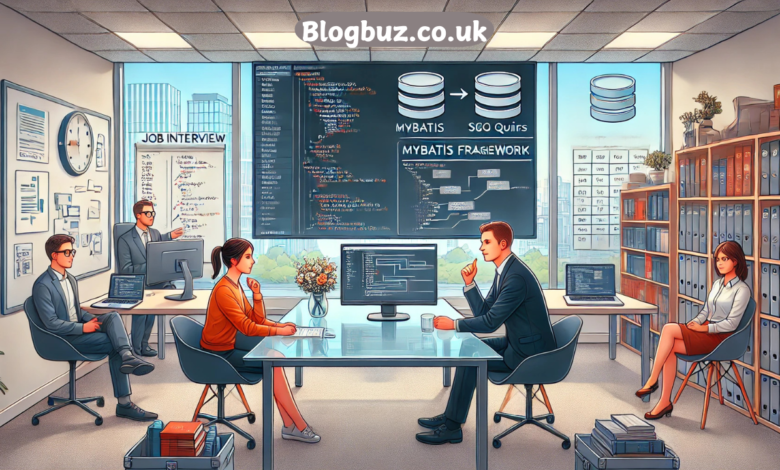
MyBatis 3.3.0 has become a popular SQL mapping framework in the Java ecosystem. Known for its straightforward approach to handling SQL queries and mapping, it allows developers greater control and flexibility when working with complex SQL commands. As a powerful tool for enterprise applications, MyBatis is often preferred over ORM frameworks like Hibernate when precision and direct SQL access are required. This post will address the most frequently requested interview questions about MyBatis 3.3.0, providing comprehensive answers to help you shine in your technical interview.
Key Topics We Will Cover
- Introduction to MyBatis 3.3.0 and how it differs from other frameworks
- Core MyBatis components and how they interact
- MyBatis’s advanced features, including dynamic SQL and caching
- Practical answers to frequently asked MyBatis interview questions.
What is MyBatis, and How Does It Differ from Hibernate?
Answer: MyBatis is a persistence framework that simplifies SQL mapping in Java applications; unlike ORM frameworks like Hibernate, which abstracts database interactions through object-relational mapping, MyBatis gives developers direct control over SQL. This approach is ideal for projects needing custom SQL statements or specific database interactions.
Comparison with Hibernate:
- Control over SQL: MyBatis allows developers to write SQL queries directly, offering more control for complex operations.
- Performance: With MyBatis, you avoid the overhead of ORM mapping, which can improve performance for complex queries.
- Use Cases: While Hibernate is ideal for applications where object mapping is preferred, MyBatis shines in applications with complex SQL requirements or legacy databases.
What Are the Core Components of MyBatis 3.3.0?
Understanding MyBatis architecture is crucial for implementing and configuring it effectively. Here are its main components:
- Configuration: The XML-based configuration file where MyBatis is set up with database connection settings, environments, and more.
- SqlSessionFactory: This factory creates SqlSession instances, which manage database connections and transactions.
- SqlSession: The primary interface for executing SQL statements and managing transactions.
- Mapper: Defines SQL queries and maps them to Java methods, acting as an interface between application code and SQL commands.
How Does MyBatis Handle SQL Injection?
Answer: MyBatis mitigates SQL injection risks by using parameterized queries. The # symbol in SQL statements represents parameter placeholders, automatically escaping special characters to prevent injection. Unlike the $ symbol, which directly substitutes input values into SQL, # provides safe handling of user input.
Example:
XML
Copy code
<!– Using # to prevent SQL injection –>
<select id=”selectUserByName” parameterType=”String” resultType=”User”>
SELECT * FROM users WHERE name = #{name}
</select>
Using # instead of $ ensures that user input is correctly escaped.
What is Dynamic SQL in MyBatis?
Dynamic SQL in MyBatis allows for the creation of flexible, adaptable queries. Using XML tags, MyBatis enables conditional SQL based on different parameters, which is invaluable in scenarios where SQL needs to adjust according to user input or business logic.
Key Dynamic SQL Tags:
- <if>: Conditionally includes SQL based on the presence or value of specific parameters.
- <choose>, <when>, <otherwise>: Similar to switch-case statements in Java, used to select one among several possible SQL segments.
- <foreach>: Used for iterating over collections, helpful for generating SQL IN clauses dynamically.
Example of dynamic SQL:
XML
Copy code
<select id=”finders” parameterType=”map” resultType=”User”>
SELECT * FROM users
<where>
<if test=”username != null”>
username = #{username}
</if>
<if test=”email != null”>
AND email = #{email}
</if>
</where>
</select>
How Do MyBatis Result Maps Work?
Answer: Result Maps are used to map SQL results to Java objects. This is especially beneficial when handling complicated mappings or nested objects that don’t match the database schema directly.
- Mapping Columns to Fields: You can define mappings for each column in the result set to corresponding fields in Java objects.
- Nested Results: MyBatis supports mapping nested objects, making it suitable for complex hierarchies.
- Customization: With Result Maps, you can override default mappings, enabling custom mapping logic.
Example:
XML
Copy code
<resultMap id=”userResultMap” type=”User”>
<id property=”id” column=”user_id”/>
<result property=”name” column=”username”/>
<result property=”email” column=”user_email”/>
</resultMap>
How Does MyBatis Handle Caching?
MyBatis includes caching mechanisms to optimize data retrieval:
- First-level Cache: Default session-level cache stores query results within a single SqlSession.
- Second-level Cache: Optional global cache across multiple sessions, configurable in the mybatis-config.xml file.
To activate second-level caching:
XML
Copy code
<cache />
How Can You Integrate MyBatis with Spring?
MyBatis can be integrated with Spring, combining MyBatis’s SQL mapping strengths with Spring’s dependency injection and transaction management.
Configuration:
- Define SqlSessionFactoryBean in Spring’s XML configuration.
- Use SqlSessionTemplate to manage MyBatis sessions in Spring.
Example:
XML
Copy code
<bean id=”sqlSessionFactory” class=” org.mybatis. Spring.SqlSessionFactoryBean”>
<property name=”dataSource” ref=”dataSource”/>
</bean>
What are MyBatis Plugins, and How Do They Work?
MyBatis plugins allow for customization and interception of core behaviors like SQL execution, parameter setting, and transaction management. Plugins enable you to add custom logging, modify queries, or handle exceptional conditions.
Example of a Logging Plugin:
java
Copy code
@Intercepts({@Signature(type = Executor.class, method = “update”, args = {MappedStatement.class, Object.class})})
public class MyBatisLoggingPlugin implements Interceptor {
@Override
public Object intercept(Invocation invocation) throws Throwable {
System. out.println(“SQL Executed: ” + invocation.getMethod().getName());
return invocation.proceed();
}
}
How Do You Optimize SQL Performance in MyBatis?
Performance tuning is critical in MyBatis, especially for large-scale applications:
- Caching: Use first and second-level caching to reduce database load.
- Batch Processing: For multiple inserts or updates, batch processing minimizes database round trips.
- Dynamic SQL Optimization: Avoid unnecessary conditions and filters in dynamic SQL statements to streamline query execution.
- Indexing: Ensure the database tables have optimized indexes, as MyBatis cannot manage this.
Common Errors in MyBatis and Debugging Tips
Error Types:
- Configuration Errors: Misconfigured XML files can lead to runtime errors.
- Mapping Issues: Mismatches between SQL results and Java properties can cause mapping failures.
Debugging Tips:
- Use MyBatis’s logging features to track SQL statements.
- Check for proper XML syntax in configuration and mapper files.
- Enable MyBatis SQL logging for error traceability.
Conclusion: Mastering MyBatis 3.3.0 for Career Growth
MyBatis 3.3.0 is a valuable skill for Java developers working with SQL-heavy applications. Understanding its components, SQL mapping, and best practices can give you a strong advantage in interviews and on the job. By mastering MyBatis’s core concepts, dynamic SQL, and caching, you’ll be well-equipped to tackle database interactions in complex applications.
You May Also Read: Starship CodeGen Agent: Revolutionizing the Software Development Landscape